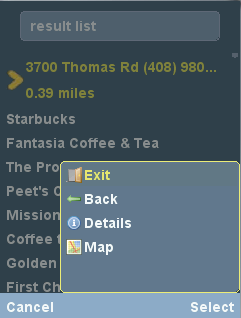
I built an infinite progress component which displays a rotating image as an animation, this considerably reduces JAR and heap space usage for an animation that looks quite similar to the one produced by a static animation (which we create from GIF). The reason for this is in the way animations work, animations have no sense of rotation, they store the change to the image (as lines) and when the image rotates the animation sees the entire image as changed and produces a "key frame". Key frames are large both in storage and in memory, to hold a 32x32 pixel animation with 8 keyframes I would need approximately 32x32x8+1024 (1024 for palette). This might not seem big, but with increase in resolution the size rises quite a bit...
Rotation is not always efficient, in fact it can be just as inefficient as a keyframe since it needs to create a new image. However, LWUIT has a special optimization on MIDP (this doesn't not apply to LWUIT on CDC) which uses the platforms built in rotation abilities for square angles (90, 180 & 270) hence removing completely the overhead of the image. This isn't enough since rotating on square angles would produce a jumpy effect, which is why I rotate once to 45 degrees and then rotate 2 images in square angles only thus producing what seems to be 8 images but only paying the cost for 2 images.
Another significant advantage is that unlike animations I can make full use of translucency since the alpha channel isn't removed in these images, this allows for flowing rotation effects.
The rotate method currently makes many assumptions and is mostly useful for square images, however it works rather nicely for all angles which is something that currently plain MIDP doesn't support.
public class InfiniteProgressIndicator extends com.sun.lwuit.Label {
private Image[] angles;
private int angle;
public InfiniteProgressIndicator(Image image) {
Image fourtyFiveDeg = image.rotate(45);
angles = new Image[] {image, fourtyFiveDeg, image.rotate(90), fourtyFiveDeg.rotate(90),
image.rotate(180), fourtyFiveDeg.rotate(180), image.rotate(270), fourtyFiveDeg.rotate(270)};
getStyle().setBgTransparency(0);
setIcon(image);
setAlignment(Component.CENTER);
}
public void initComponent() {
getComponentForm().registerAnimated(this);
}
public boolean animate() {
angle++;
setIcon(angles[Math.abs(angle % angles.length)]);
return true;
}
}
Resource editor works on Windows regardless of an IDE. So it will work for Eclipse users too and also for your UI designers.
ReplyDeleteHi Shai, I tried to dynamically replace this component of yours, once my thread returns. however no success. Can you explain how one should implement such a callback to replace the progressindicator with (like in my use case) a plain label with image on a Form?
ReplyDeleteI tried creating new label, deregisteranimation&setting icon on indicator, remove component and all failed (indicator just stops rotating, but is never replaced...)
Thxs a lot ben
Thanks the revalidate did the trick.
ReplyDeleteUhm, maybe a silly question but why the splitting into the 0,90,180,270 and the 45,135,225,315 degrees ?
ReplyDeleteAs I explained in the post MIDP (hence LWUIT as well) supports a special case for square angles (divisions of 90 degrees) which makes these very efficient and fast. As a result LWUIT automatically identifies 90 degree angles and creates a rotation image that doesn't occupy additional RAM...
ReplyDeleteSo I have what seems like 8 images but taking up as much RAM as 2 images.
Oups, sorry, I missed that, hopped straight to the code.
ReplyDeleteHi
ReplyDeletei tested this animation on revision 242 and it worked fine
but now same animation on revision265 looks jumpy (like frames are miss ordered or somthing)
can u check? what has changed?
I'm using this with the latest sources without a problem.
ReplyDeletehmmm
ReplyDeletewell im also using latest code and when the image is rotated 90 it also get mirrored
in your code u dont have delay in the animate method
i placed a delay (not a real delay but a check for delta time has passed)
and only then i increase the angle
maybe u see it too fast to notice?
BR
shai
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeletehi shai, first of all this dialog looks great :D
ReplyDeletebut I have a question regarding it, where and how do I change the used theme for it ? For example it has a yellow border which i'd like to change to another color... where should i be doing that ?
Please spend some time reading the tutorial/developer guide regarding themes.
ReplyDeleteI tested the animation of progress indicator(in my application). But when change the configuratin of mobile it leads an exception as IllegalArgumentException.
ReplyDeletePls, give me ur valuable suggestion for this--------
Pls---------------